Next.js
Framer Motion
Tailwind
Mekmo is a digital agency which creates visually impressive websites and software. They needed a website to reflect this so I planned and built the entire site using Framer Motion and React.
The site is filled with many small interactions used to make the site come alive. Many of these are handled easily using Framer motion such as the intro elements when scrolling through the page:
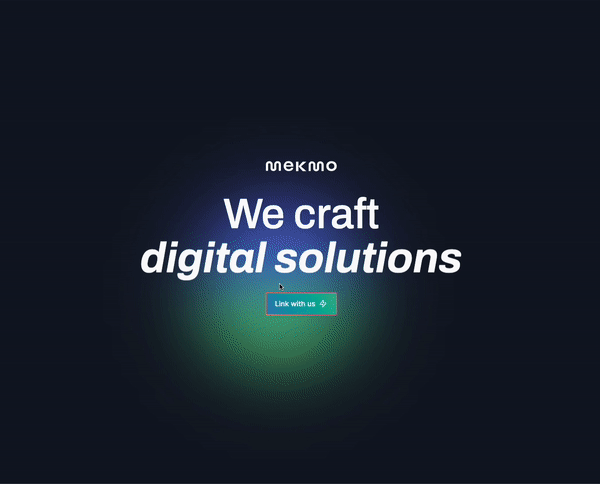
Other interactions such as the card hover effects were not so simple. They leveraged parts of Framer motion to handle the animation itself while the logic was written by me:
const handleMouseMove: MouseEventHandler<HTMLDivElement> = useCallback(
(e) => {
if (!el.current) return
// Animation should only work on desktop screen sizes.
if (window.innerWidth <= 768) return
// Get the position of the cursor within the element.
const rect = el.current.getBoundingClientRect()
const x = e.clientX - rect.left
const y = e.clientY - rect.top
const xPercent = (x - rect.width / 2) / (rect.width / 2)
const yPercent = (y - rect.height / 2) / (rect.height / 2)
// Use framer motion to animate the card's rotation
// based on the cursor position.
animation.start({
rotateY: -5 * xPercent,
rotateX: 5 * yPercent,
transition: {
ease: 'easeOut',
},
})
},
[animation]
)
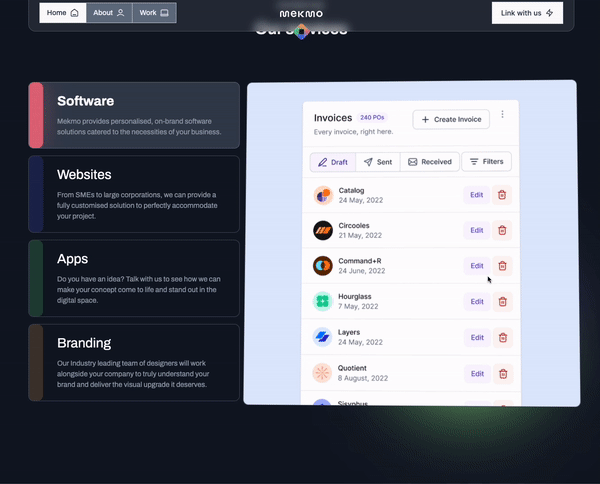
My responsibilities
- Planning and building the site
- Implementing new features or changing existing components
- Deploying the site